Systems Programming (XMUT)
Exercise 0: Installing Code::Blocks and the first project

Due 8 September 7 pm
Overview
This lab introduces you to the software that you will be using for your assignments, gets you to familiarise yourself with creating and running a very simple c program.
Resources and links
To Submit
Development Workflow
The development process is pretty simple and can be summarised into four major steps:
- Edit source file using Code::Blocks IDE.
- Compile source file using Code::Blocks IDE.
- If bugs or warnings are present, debug and go to step 1.
- Execute executable output. If bugs are present, debug and go to step 1.
In Sections 1--4, we will go through each and every step of the workflow to get yourself ready to work on the first set of programming exercises.
1) Creating a project with Code::Blocks IDE
- Click on
File → New → Project
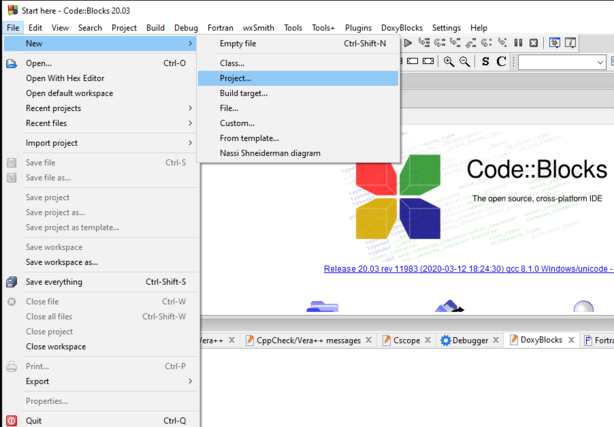
- Select Empty Project from the project category and then click on the Go button
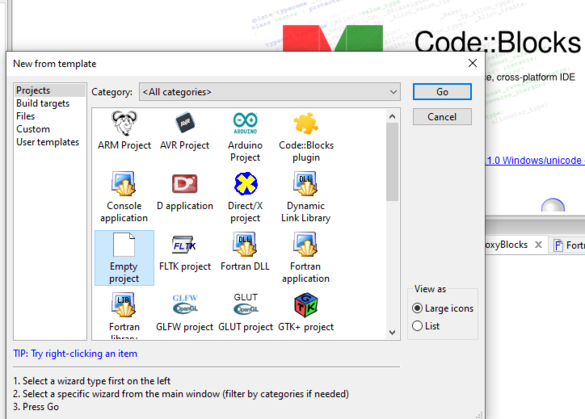
- On the Welcome message dialogue box, check the Skip this page next time checkbox. And click on the Next button.
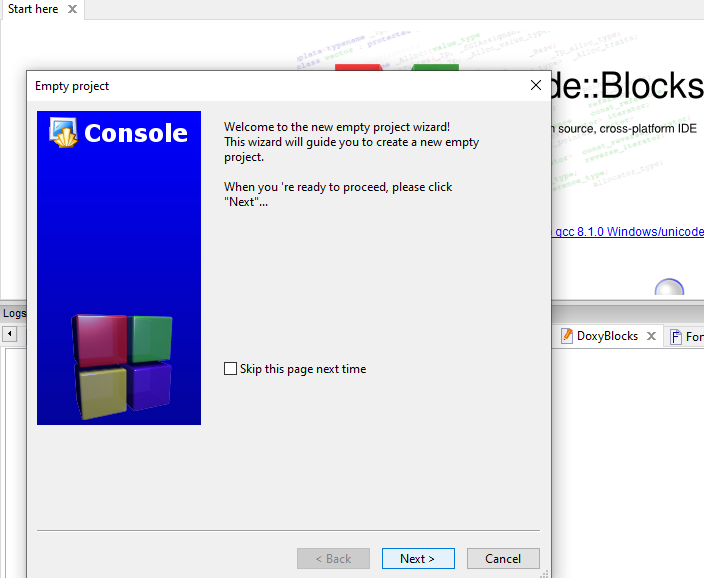
- On the next wizard screen, enter your Project title and path where to store your project. Enter any title of your project suppose HelloWorld. Choose the file path where you want to store your C files. For choosing a file path click on the ... button. And click on the Next button. It does not matter where on your computer you put this folder, in this example, the directory C:\Users\nekooei\OneDrive\Desktop\NWEN241\ is selected as the root directory.
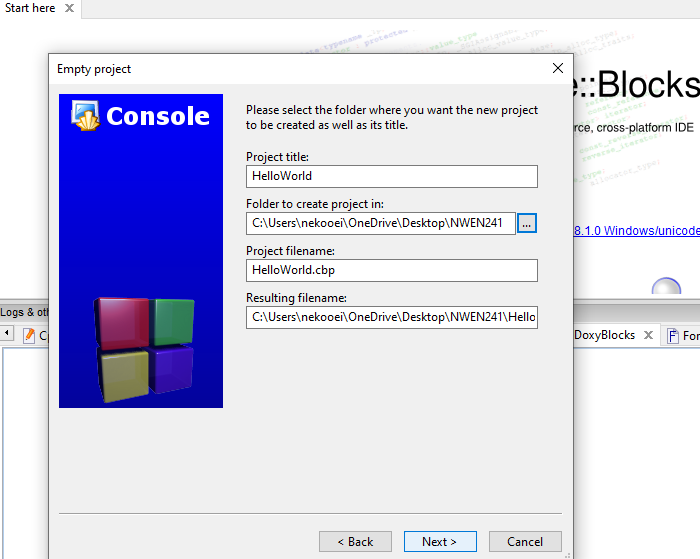
- The next screen asks you to choose a C/C++ compiler. If you have installed the GNU C/C++ compiler then you need not perform any action on this screen simply click on the Finish button. If you don't have a GNU C/C++ compiler download it before preceding the next step.
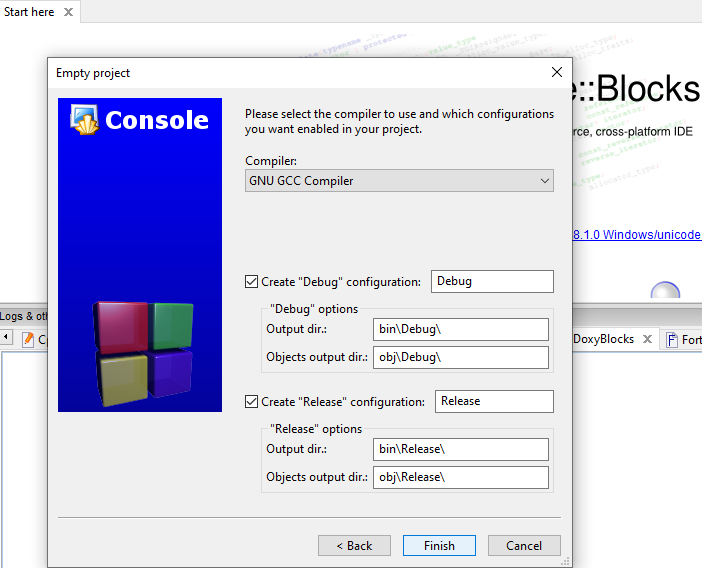
- Create a new file. Click on
File → New → File
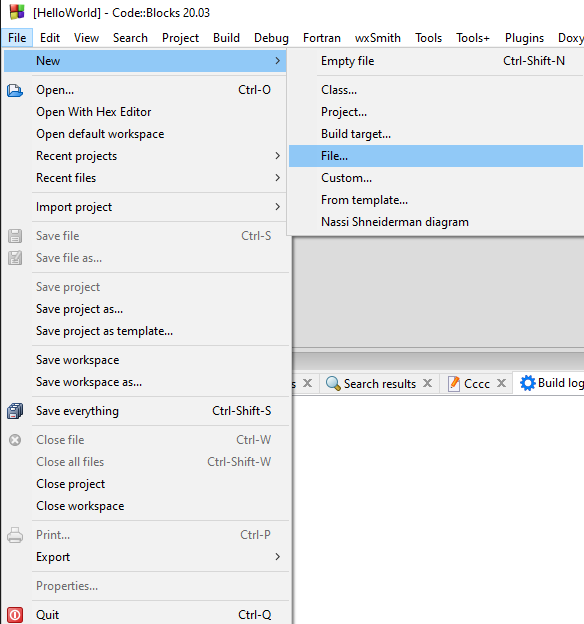
- From the New form template window select C/C++ source and click the Go button
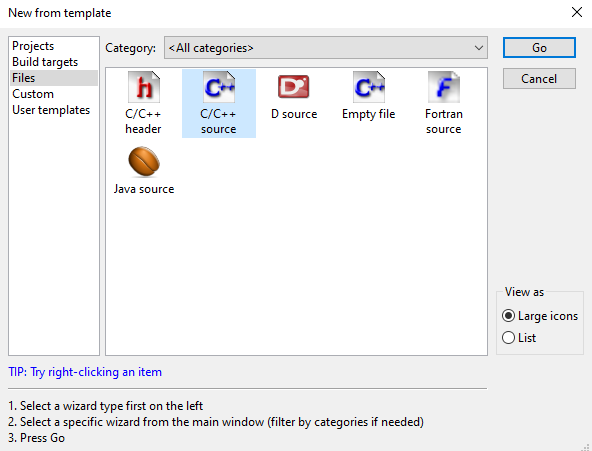
- If you see a welcome message, click next to skip the welcome message. Make sure you have checked the Skip this next time checkbox if you do not want to see this welcome message again.
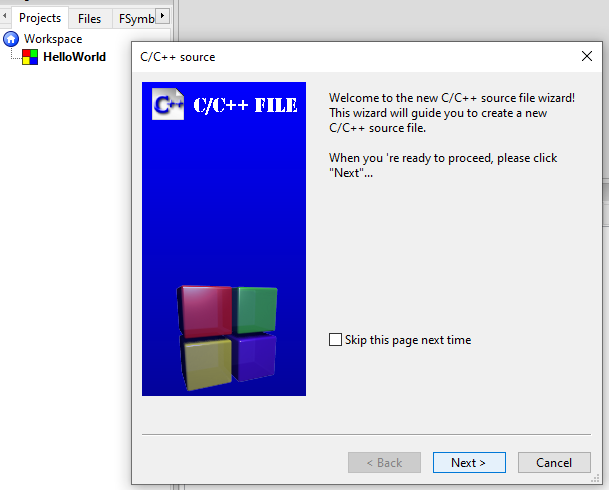
- Next, select your language from the C source window and click the Next button.
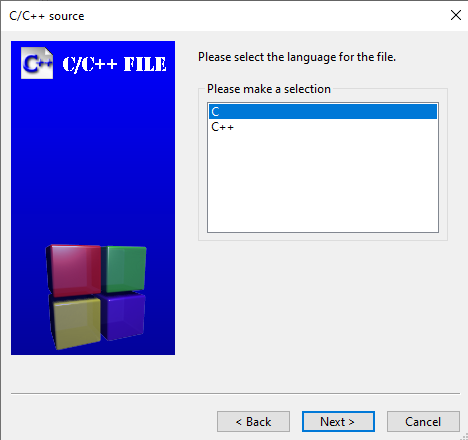
- Give a name to your file and specify the location. For choosing a file path click on the ... button. Type "hello.c" as the name of the C file and click on the Save button.
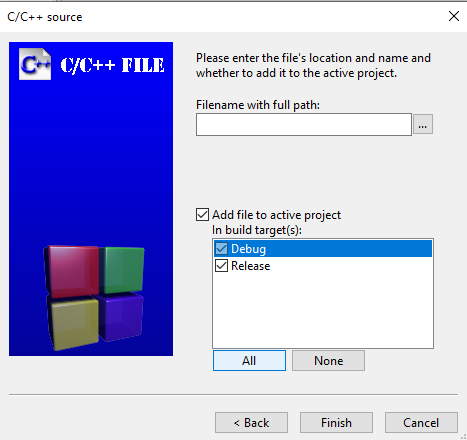
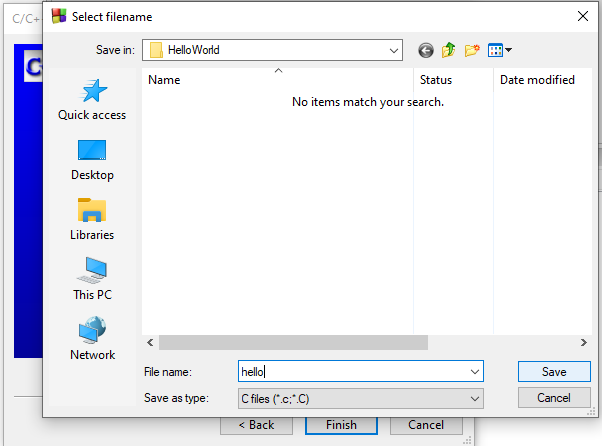
- click Finish.
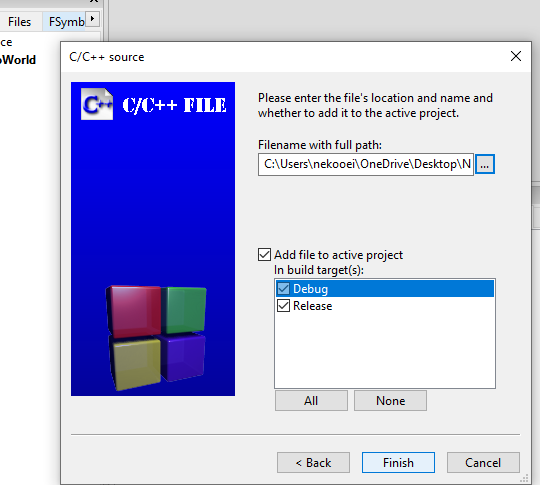
2) Editing Source Code
Congratulations you have created your first C/C++ project in Code::Blocks. But where do I write my first C/C++ program as no editor opens after the last step? To open the code editor, navigate to the workspace area and click on the
+ symbol present before Sources. That will expand the sources folder. Now double click on hello.c file to open the code editor.
Type the following simple C program in the text editor as-is:
#include <stdio.h>
int main(void)
{
printf("Hello, world\n")
}
(The above source code has intentional errors. Do not fix them at this time.)
3) Compiling
To run any C/C++ program click on
Build → Build and run
or simply hit
F9
from your keyboard.
4) Debugging
If the compiler generates error messages, that means that your source code has bugs that you need to fix. To debug your source code, read and understand the error messages carefully.
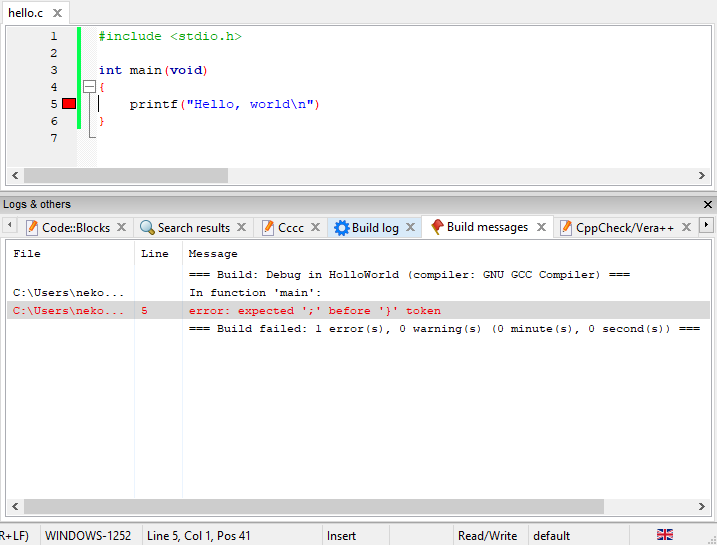
In the above example, the error says that there is a missing semi-colon (
;
) before the parenthesis. It even suggests where the semi-colon should be inserted. This gives you a good clue on how to fix the code. Basically, we just have to insert a semi-colon at the end of line 5 as follows:
#include <stdio.h>
int main(void)
{
printf("Hello, world\n");
}
Edit your source code to fix the problem and compile it again. As you can see, the compiler did not generate any error or warning message. That means that it was successful, and it generated an executable file. The Code::Blocks also shows the output screen.

Submit your code
hello.c
. Make sure that you submit the
.c
file, not the
.exe
file: the
.exe
file is the compiled version which the markers cannot read!